Java文件压缩操作
原文:http://hello-nick-xu.iteye.com/blog/2002613
在web开发过程中,进行文件的压缩传输是一种常见的需求。比如一种场景:用户需要下载定时生成的报表,我们需要先对报表文件进行压以方便用户的下载,并减少文件的存储空间。
事实上,JDK已经提供了文件压缩/解压缩的支持,可以生成zip/gzip的压缩格式,并且支持支持“校验和”以检查压缩文件的完整性。通常会使用CRC(循环冗余校验)算法进行校验。
遗憾的是,JDK的文件压缩功能无法处理文件名称包含中文的问题。为此,我们需要用到ant.jar第三方开源支持包。
如下所示代码,采用Junit 4进行压缩功能的测试:
- /*
- * Copyright (c) 2014, hello_nick_xu@foxmail.com, All rights reserved.
- */
- package com.test.zip;
- import java.io.BufferedInputStream;
- import java.io.BufferedOutputStream;
- import java.io.File;
- import java.io.FileInputStream;
- import java.io.FileOutputStream;
- import java.util.zip.CRC32;
- import java.util.zip.CheckedOutputStream;
- import org.apache.tools.zip.ZipEntry;
- import org.apache.tools.zip.ZipOutputStream;
- import org.junit.Test;
- /**
- * 功能简述: 进行文件压缩功能测试
- * @author Nick Xu
- */
- public class ZipTest {
- //源文件夹
- private final String sourceDirStr = "E:/source";
- //目标文件
- private final String zipPath = "E:/dest.zip";
- //缓冲区大小设置
- private static final int BUFFER_SIZE = 1024;
- /**
- * 功能简述: 普通压缩测试
- * @throws Exception
- */
- @Test
- public void testZip() throws Exception {
- // zip输出流:使用缓冲流进行了包装
- ZipOutputStream zipOut = new ZipOutputStream(
- new BufferedOutputStream(
- new FileOutputStream(zipPath), BUFFER_SIZE));
- // 得到源文件夹下的文件列表
- File sourceDirectory = new File(sourceDirStr);
- File[] files = sourceDirectory.listFiles();
- byte[] bs = new byte[BUFFER_SIZE]; // 缓冲数组
- int value = -1; //文件是否结束标记
- for (File file : files) { // 遍历所有的文件
- zipOut.putNextEntry(new ZipEntry(file.getName())); // 存入文件名称,便于解压缩
- //文件读写
- BufferedInputStream bfInput = new BufferedInputStream(
- new FileInputStream(file), BUFFER_SIZE);
- while ((value = bfInput.read(bs, 0, bs.length)) != -1) {
- zipOut.write(bs, 0, value);
- }
- bfInput.close(); //关闭缓冲输入流
- }
- //关闭输出流
- zipOut.flush();
- zipOut.close();
- }
- /**
- * 功能简述: 文件压缩测试,获取校验和
- * @throws Exception
- */
- @Test
- public void testZipWithCheckSum() throws Exception {
- //被校验的输出流:使用CRC32校验算法
- CheckedOutputStream checkedOutput = new
- CheckedOutputStream(new BufferedOutputStream(
- new FileOutputStream(zipPath), BUFFER_SIZE),new
- CRC32());
- //使用ZipOutputStream进行包装
- ZipOutputStream zipOut = new ZipOutputStream(checkedOutput);
- // 得到文件名称列表
- File sourceDirectory = new File(sourceDirStr);
- File[] files = sourceDirectory.listFiles();
- // 读写文件
- byte[] bs = new byte[BUFFER_SIZE];
- int value = -1;
- for (File file : files) {
- BufferedInputStream bfInput = new BufferedInputStream(
- new FileInputStream(file), BUFFER_SIZE);
- zipOut.putNextEntry(new ZipEntry(file.getName()));
- while ((value = bfInput.read(bs, 0, bs.length)) != -1) {
- zipOut.write(bs, 0, value);
- }
- bfInput.close();
- }
- zipOut.flush();
- zipOut.close();
- //获取校验和:用于校验文件的完整性
- long checkSum = checkedOutput.getChecksum().getValue();
- System.out.println("checkSum : " + checkSum);
- }
- }
完整项目功能,参见附件
标签: java文件压缩
日历
个人资料
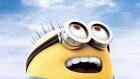
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: