算法-二叉树-最大距离
package com.jiucaiyuan.net.algrithm.tree; /** * 树形dp套路 * <p> * 树形dp套路使用前提: * 如果题目求解目标是S规则,则求解流程可以定成每一个节点为头节点的子树 * 在S规则下的每个答案,并且最终答案一定在其中 * <p> * 案例题 * 【题目】二叉树节点间的最大距离 * 从二叉树的节点a出发,可以向上或者向下走,但是沿途节点只能经过一次, * 到达节点b时路径上节点的个数叫做a到b的距离,那么二叉树任意两个节点 * 之间都有距离,求整棵树上的最大距离 * 【思路】 * 划分为三种情况: * 1.头结点参与,最大可能是左树高+1+右树高 * 2.头结点不参与,左树最大距离 * 3.头结点不参与,右树最大距离 * 从三种中选择最大值,就是整棵树的最大距离 * * @Author jiucaiyuan 2022/5/6 23:06 * @mail services@jiucaiyuan.net */ public class MaxDistanceInTree { public static class Info { public int maxDistance; public int height; public Info(int dis, int h) { this.maxDistance = dis; this.height = h; } } /** * 取得以head为头结点的整棵树最大距离 * * @param head 树的头结点 * @return head树的最大距离 */ public static int maxDistance(Node head) { return process(head).maxDistance; } /** * 返回以head为头结点的整棵树的信息 * * @param head * @return */ private static Info process(Node head) { if (head == null) { return new Info(0, 0); } //向左子树要信息 Info left = process(head.left); //向右子树要信息 Info right = process(head.right); //最大距离根据头结点是否参与分成三种情况,三种情况最大值,是本结点的最大距离 int dis = Math.max(Math.max(left.maxDistance, right.maxDistance), left.height + 1 + right.height); //树的高度是左子树和右子树较大的+1 int h = Math.max(left.height, right.height) + 1; return new Info(dis, h); } }
日历
个人资料
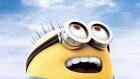
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: