算法-二叉树-判断满二叉树
package com.jiucaiyuan.net.algrithm.tree; /** * <pre> * 【问题】判断一棵树是满二叉树(Full Binary Tree) * 【概念】满二叉树:一个二叉树,如果每一个层的结点数都达到最大值,则这个二叉树就是满二叉树。 * 也就是说,如果一个二叉树的深度为K,且结点总数是(2^k) -1 ,则它就是满二叉树。 * (一棵满二叉树的每一个结点要么是叶子结点,要么它有两个子结点,但是反过来不成立, * 因为完全二叉树也满足这个要求,但不是满二叉树) * 【思路】获得二叉树的深度k及节点个数,然后判断节点个数是否等于(2^k) -1 * </pre> * * @Author jiucaiyuan 2022/4/10 17:00 * @mail services@jiucaiyuan.net */ public class IsFullBinaryTree { /** * 递归套路求解 * * @param root */ public static boolean isFullBinaryTree(Node root) { if (root == null) { return true; } Info rootInfo = f(root); return rootInfo.nodes == ((1 << rootInfo.height) - 1); } /** * 从左子树获取信息+右子树获取信息,加工出当前子树的信息 * @param x * @return 返回以x为跟的树高度和节点个数 */ public static Info f(Node x) { if (x == null) { return new Info(0, 0); } Info leftInfo = f(x.left); Info rightInfo = f(x.right); int height = Math.max(leftInfo.height, rightInfo.height) + 1; int nodes = leftInfo.nodes + rightInfo.nodes + 1; return new Info(height, nodes); } static class Info { int height; int nodes; public Info(int h, int n) { height = h; nodes = n; } } }
日历
个人资料
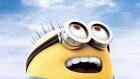
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: