算法-链表-找到单链表入环节点
问题
判断一个单链表是否存在环,如果存在返回入环节点
要求
时间复杂度O(N),空间复杂度O(1)
方案
快慢指针:从头结点开始,快指针一次走两步,满指针一次走一步,如果这两个指针相遇后,快指针回到头结点,两个指针一起开始走,每次走一步,再次相遇的节点即是入环节点
代码
package com.jiucaiyuan.net.algrithm.linked; import java.util.HashSet; import java.util.Set; /** * 【问题】:判断一个单链表是否存在环,如果存在返回入环节点 * 【方法】快慢指针:从头结点开始,快指针一次走两步,满指针一次走一步, * 如果这两个指针相遇后,快指针回到头结点,两个指针一起开始走, * 每次走一步,再次相遇的节点即是入环节点 * * @Author jiucaiyuan 2022/4/7 23:12 * @mail services@jiucaiyuan.net */ public class FindLoopNode { /** * 确实简洁 * @param head * @return */ public static ListNode getLoopNode(ListNode head){ if(head == null || head.next == null || head.next.next == null){ return null; } ListNode n1 = head.next; ListNode n2 = head.next.next; while(n1!= n2) { if (n2.next == null || n2.next.next == null) { return null; } n1 = n1.next; n2 = n2.next.next; } n2 = head; while (n1 != n2){ n1 = n1.next; n2 = n2.next.next; } return n1; } public static ListNode entryNodeOfLoop(ListNode pHead) { if (pHead == null) { return null; } ListNode fast = pHead; ListNode low = pHead; boolean second = false; while (low != null && fast != null && fast.next != null) { if (fast == low) { if (second) { return fast; } if (fast != pHead && low != pHead) { fast = pHead; second = true; continue; } } low = low.next; if (second) { fast = fast.next; } else { fast = fast.next.next; } } //如果快慢指针有一个为null,则不存在环 return null; } public static void print(ListNode head) { Set<ListNode> set = new HashSet<>(); System.out.println("-------------------------"); while (head != null) { if(!set.contains(head)){ set.add(head); }else{ System.out.print("\t入环节点="+head.val); break; } System.out.print("\t"+head.val); head = head.next; } System.out.println(); System.out.println("-------------------------"); } public static void main(String[] args) { int[] arrays = {1, 2, 3, 4, 15,20,21,43,25,8,5,40}; int entryNodeValue = 21; // int[] arrays = {0}; ListNode head = new ListNode(arrays[0]); ListNode tail = null; ListNode entryNode = null; if (arrays.length > 1) { ListNode tmp = new ListNode(arrays[1]); head.next = tmp; for (int i = 2; i < arrays.length; i++) { tail = new ListNode(arrays[i]); if (arrays[i] == entryNodeValue) { entryNode = tail; } tmp.next = tail; tmp = tmp.next; } } tail.next = entryNode; print(head); System.out.println("找到的入环节点是:"+entryNodeOfLoop(head)); } }
输出结果
-------------------------
1 2 3 4 15 20 21 43 25 8 5 40 入环节点=21
-------------------------
找到的入环节点是:ListNode{val=21}
Process finished with exit code 0
日历
个人资料
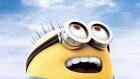
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: