算法-堆排序扩展排序几乎有序数组
package com.jiucaiyuan.net.algrithm.sort; import java.util.PriorityQueue; /** * <pre> * 堆排序扩展 * 一个几乎有序的数组, * 几乎有序是指,如果把数组排好顺序的话, * 每个元素移动的距离可以不超过k,这个k相对于数组来说比较小, * 请选择合适的排序算法针对这个数组进行排序 * * 时间复杂度O(N*logk) 空间复杂度O(k) * * </pre> * Created by jiucaiyuan on 2022/3/31. */ public class HeapSortExtension { /** * 堆排序算法 * * @param arr * @return */ public static void sortedArrDistanceLessK(int[] arr, int k) { //默认是小根堆 PriorityQueue<Integer> heap = new PriorityQueue<>(); //把k个数放入小根堆 int index = 0; for (; index <= Math.min(arr.length, k); index++) { heap.add(arr[index]); } //把数组剩下的数逐个放入小根堆,并逐个弹出小根堆头结点 int i = 0; for (; index < arr.length; i++, index++) { heap.add(arr[index]); arr[i] = heap.poll(); } //如果堆不为空,全部弹出 while (!heap.isEmpty()) { arr[i++] = heap.poll(); } } public static void main(String[] args) { int[] arr = {12, 5, 3, 7, 3, 8, 4, 3, 2, 2, 6, 8, 6, 0, 9, 5, 3}; print(arr); sortedArrDistanceLessK(arr, 3); print(arr); } private static void print(int[] arr) { if (arr == null) { return; } for (int a : arr) { System.out.print(a + "\t"); } System.out.println(); } }
日历
个人资料
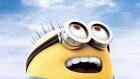
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: