算法-43.字符串相乘
package com.jiucaiyuan.net.question; /** * @Author jiucaiyuan 2022/3/22 21:59 * @mail services@jiucaiyuan.net */ public class TwoStrMultiply { /** * v2 leeCode上学的,确实方法好 * https://leetcode-cn.com/problems/multiply-strings/solution/zi-fu-chuan-xiang-cheng-by-leetcode-solution/ * * 方法一的做法是从右往左遍历乘数,将乘数的每一位与被乘数相乘得到对应的结果,再将每次得到的结果累加, * 整个过程中涉及到较多字符串相加的操作。如果使用数组代替字符串存储结果,则可以减少对字符串的操作。 * 令 m 和 n 分别表示 num1和 num2的长度,并且它们均不为 0,则 num1 和 num2 的乘积的长度为 * m+n−1 或 m+n。简单证明如下: * * case1 如果 num1和 num2都取最小值,则 num1=10^(m−1),num2=10^(n−1), * num1×num2=10^(m+n−2) ,乘积的长度为 m+n-1; * case2 如果 num1 和 num2都取最大值,则 num1=10^m − 1, num2=10^n − 1 , * num1×num2 =10^(m+n) − 10^m − 10^n + 1, 乘积显然小于 10^(m+n)且大于 10^(m+n-1) , * 因此乘积的长度为 m+nm+n。 * * 由于 num1 和 num2的乘积的最大长度为 m+n,因此创建长度为 m+n 的数组 ansArr 用于存储乘积。 * 对于任意 0≤i<m 和 0≤j<n,num1[i] * num2[j] 的结果位于 ansArr[i+j+1], * 如果 ansArr[i+j+1]≥10,则将进位部分加到 ansArr[i+j]。 * 最后,将数组 ansArr 转成字符串,如果最高位是 00 则舍弃最高位。 * * @param num1 * @param num2 * @return */ public static String multiply2(String num1, String num2) { if (num1.equals("0") || num2.equals("0")) { return "0"; } int m = num1.length(); int n = num2.length(); //存放结果 int[] ansArr = new int[m + n]; for (int i = m - 1; i >= 0; i--) { int x = num1.charAt(i) - '0'; for (int j = n - 1; j >= 0; j--) { int y = num2.charAt(j) - '0'; //num1[i] * num2[j] 的结果位于 ansArr[i+j+1] ansArr[i + j + 1] += x * y; } } //逐个检查,有大于10的,处理进位 for (int i = m + n - 1; i > 0; i--) { ansArr[i - 1] += ansArr[i] / 10; ansArr[i] %= 10; } //如果第一位是0,则舍弃 int index = ansArr[0] == 0 ? 1 : 0; StringBuffer ans = new StringBuffer(); while (index < m + n) { ans.append(ansArr[index]); index++; } return ans.toString(); } /** * v1 数字太大会越界 * @param num1 * @param num2 * @return */ public static String multiply(String num1, String num2) { int result = 0; if (num1.equals("0") || num2.equals("0")) { return String.valueOf(result); } char[] str1Arr = num1.toCharArray(); char[] str2Arr = num2.toCharArray(); int weight1 = 1; for (int i = str1Arr.length - 1; i >= 0; i--) { if(i != str1Arr.length - 1 ){ weight1 *= 10; } int weight2 = 1; for (int j = str2Arr.length - 1; j >= 0; j--) { if(j != str2Arr.length - 1 ){ weight2 *= 10; } int product = ((str1Arr[i] - '0') * (str2Arr[j] - '0')); int weight = weight1 * weight2; result += weight * (product % 10) + (product / 10) * weight * 10; } } return String.valueOf(result); } public static void main(String[] args) { String str2 = "123"; String str1 = "3"; System.out.println(multiply(str1, str2)); str2 = "200"; str1 = "200"; System.out.println(multiply(str1, str2)); str2 = "21"; str1 = "21"; System.out.println(multiply(str1, str2)); str2 = "210"; str1 = "210"; System.out.println(multiply(str1, str2)); str2 = "456"; str1 = "654"; System.out.println(multiply(str1, str2)); str2 = "5102381023812"; str1 = "200123123"; System.out.println(multiply(str1, str2)); System.out.println(multiply2(str1, str2)); } }
日历
个人资料
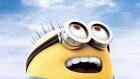
diaba 寻求合作请留言或联系mail: services@jiucaiyuan.net
链接
最新文章
存档
- 2025年4月(17)
- 2025年3月(25)
- 2025年2月(20)
- 2025年1月(2)
- 2024年10月(1)
- 2024年8月(2)
- 2024年6月(4)
- 2024年5月(1)
- 2023年7月(1)
- 2022年10月(1)
- 2022年8月(1)
- 2022年6月(11)
- 2022年5月(6)
- 2022年4月(33)
- 2022年3月(26)
- 2021年3月(1)
- 2020年9月(2)
- 2018年8月(1)
- 2018年3月(1)
- 2017年3月(3)
- 2017年2月(6)
- 2016年12月(3)
- 2016年11月(2)
- 2016年10月(1)
- 2016年9月(3)
- 2016年8月(4)
- 2016年7月(3)
- 2016年6月(4)
- 2016年5月(7)
- 2016年4月(9)
- 2016年3月(4)
- 2016年2月(5)
- 2016年1月(17)
- 2015年12月(15)
- 2015年11月(11)
- 2015年10月(6)
- 2015年9月(11)
- 2015年8月(8)
分类
热门文章
- SpringMVC:Null ModelAndView returned to DispatcherServlet with name 'applicationContext': assuming HandlerAdapter completed request handling
- Mac-删除卸载GlobalProtect
- java.lang.SecurityException: JCE cannot authenticate the provider BC
- MyBatis-Improper inline parameter map format. Should be: #{propName,attr1=val1,attr2=val2}
- Idea之支持lombok编译
标签
最新评论
- logisqykyk
Javassist分析、编辑和创建jav... - xxedgtb
Redis—常见参数配置 - 韭菜园 ... - wdgpjxydo
SpringMVC:Null Model... - rllzzwocp
Mysql存储引擎MyISAM和Inno... - dpkgmbfjh
SpringMVC:Null Model... - tzklbzpj
SpringMVC:Null Model... - bqwrhszmo
MyBatis-Improper inl... - 乐谱吧
good非常好 - diaba
@diaba:应该说是“时间的度量依据”... - diaba
如果速度增加接近光速、等于光速、甚至大于...
最新微语
- 在每件事情上花费的东西,就是生命的一部分,而我们花费的这些东西要求立即得到回报,或者在一个长时间以后得到回报。
2025-01-23 15:46
- 诺曼·文森特说:“并不是你认为自己是什么样的人,你就是什么样的人。但是你的思想是什么样,你就是什么样的人。”
2025-01-23 15:44
- 从今天起,做一个幸福的人。喂马,砍柴,(思想)周游世界
2022-03-21 23:31
- 2022.03.02 23:37:59
2022-03-02 23:38
- 几近崩溃后,找到解决方法,总是那么豁然开朗!所以遇到问题要坚持!
2018-07-18 10:49
发表评论: